The Problem
The new Version 5 of the Cloudflare Terraform and OpenTofu Provider was completely rewritten, which results in a lot of breaking changes such as renamed resources and attributes.
While there is a guide for upgrading to the Cloudflare provider v5 which includes a GritQL-Automation that does this renaming automatically, this Cloudflare-provided method is not suitable for resources that are located inside terraform-modules as stated here.
This means, that for setups where all or most of your Cloudflare resources are located inside modules, you’ll have to manually create a lot of “import” and “state rm” commands to do the migration. For the 317 resources currently in my state, this would be a very time-consuming process.
If you just update to the new version 5 of the provider without doing manual migrations inside modules, you’ll see an error message like this:
│ Error: Unable to Read Previously Saved State for UpgradeResourceState
…
│ AttributeName("data"): invalid JSON, expected "{", got "["
A script for automated imports
To fix this problem and upgrade to Cloudflare provider v5 with minimal manual work, I created this script, that creates the import and delete commands. The Script will generate two other scripts, one for deleting the resources from the state and one for re-importing them under the new schema.
The Script currently only works for the cloudflare_record resource which was renamed to cloudflare_dns_record, but it can probably be adapted to fit other resources as well.
#!/bin/bash
# config
OLD_RES_NAME="cloudflare_record"
NEW_RES_NAME="cloudflare_dns_record"
# delete/create files
if [ -f state_deletes.sh ]; then
cp state_deletes.sh state_deletes.sh.bak
fi
if [ -f state_imports.sh ]; then
cp state_imports.sh state_imports.sh.bak
fi
echo "set -e" > state_deletes.sh
echo "set -e" > state_imports.sh
chmod +x state_deletes.sh
chmod +x state_imports.sh
# get existing records in modules and count
resources=$(terraform state list | grep module | grep $OLD_RES_NAME)
count=$(echo "$resources" | wc -l)
echo "## Found $count resources to process."
# loop over resources, get details and write scripts
current=0
for line in $resources; do
current=$((current + 1))
echo "## Processing resource $current of $count: $line"
echo "echo '## Processing resource $current of $count: $line'" >> state_deletes.sh
echo "terraform state rm '$line'" >> state_deletes.sh
import_line=$(echo "$line" | sed "s~$OLD_RES_NAME~$NEW_RES_NAME~g")
import_id=$(terraform state show "$line" | grep -E "zone_id| id " | tac | cut -d "=" -f2 | tr -d " " | tr -d '"' | tr "\n" "/" | sed 's~/$~~g')
echo "echo '## Processing resource $current of $count: $import_line'" >> state_imports.sh
echo "sleep 2; terraform import '$import_line' '$import_id'" >> state_imports.sh # sleep to avoid rate limiting, as imports trigger refreshs with a lot of api-requests.
done
echo "## Processing complete."
How to use the Script
1. Preparation and Backup
Make sure you have a local terraform install which has access to your statefile.
If you tried any state migration before (e.g. running the grit script from the official guide), revert to the original statefile. Migrations you might already did in the terraform code itself are OK and don’t have to be reverted.
⚠️ Use the command below to create a backup of your state before modifications!
terraform state pull > state_before_cf_v5.tfstate
Also, ensure your Cloudflare Provider version is fixed to the old 4.x (as shown below) and finally run “terraform init”.
terraform {
required_providers {
cloudflare = {
source = "cloudflare/cloudflare"
version = "~> 4"
}
}
}
2. Running the script generator
Copy the code for the “generate_tf_commands.sh” from the start of this article and create the file in your terraform workdir. Make it executable and run the script as shown below. Afterwords, you will find 2 new files in your workdir named “state_deletes.sh” and “state_imports.sh”.
chmod +x generate_tf_commands.sh
./generate_tf_commands.sh
3. Deleting from the state
Run the generated delete script. This process might take a while to complete.
./state_deletes.sh
4. Upgrade to Cloudflare provider v5
Change your provider configuration as stated below to upgrade to version 5 and upgrade the provider.
terraform {
required_providers {
cloudflare = {
source = "cloudflare/cloudflare"
version = "~> 5"
}
}
}
terraform init -upgrade
5. Follow the official upgrade guide to change your code
Follow the official upgrade guide to change the names and attributes in your code. To do so, make sure, you have grit installed. Afterwords, run the commands below. Make sure, to also run this on all modules you have, that currently use Cloudflare resources.
# Changing attributes
grit apply github.com/cloudflare/terraform-provider-cloudflare#cloudflare_terraform_v5
# Changing names
grit apply github.com/cloudflare/terraform-provider-cloudflare#cloudflare_terraform_v5_resource_renames_configuration
If you also have cloudflare resources outside modules in the root of your terraform code, you also need to run:
# Acquiring the statefile
terraform state pull > state_to_edit.tfstate
# Doing the state migrations for resources outside modules - this will take a while
grit apply --force github.com/cloudflare/terraform-provider-cloudflare#cloudflare_terraform_v5_attribute_renames_state state_to_edit.tfstate
grit apply --force github.com/cloudflare/terraform-provider-cloudflare#cloudflare_terraform_v5_resource_renames_state state_to_edit.tfstate
# push edited state, ignoring unchanged serial
terraform state push -force state_to_edit.tfstate
At this point, you’ll also have to treat all other manual migrations, which are explained in the upgrade guide. The Script currently only works for the cloudflare_record resource which was renamed to cloudflare_dns_record, but it can probably be adapted to fit other resources as well.
If you have resources in your code that were replaced without requiring migration, like cloudflare_zone_settings_override, you’ll have to delete them from your state. You can use this snippet to do so, replacing cloudflare_zone_settings_override with the resource of your choice:
terraform state list | grep cloudflare_zone_settings_override | sed 's/"/\\"/g' | xargs -i bash -c "terraform state rm '{}'"
6. Re-Import the resources in the new version
Run the generated import script. This process again will take a while to complete, depending on the number of resources that need changing.
By default, the scripts include a “sleep 2” between all imports, as each import triggers a state refresh of dependent resources which results in a lot of Cloudflare API-Requests which again leads to failures. In my case, a 2-second pause was just enough to get through my 300 resources on the Cloudflare free-plan, while 1s was too low. You may have to adjust this depending on your Cloudflare Plan and number of resources.
./state_imports.sh
7. Finish: Run terraform plan
Finally, run terraform plan and check if it now can complete without errors. If you have other resources in modules than cloudflare_record, you’ll need to adjust the script to fit those. Feel free to reach out at git [at] pascal-hoehnel.de if you want me to add your solution to this article!
FAQ
Can I just upgrade from Cloudflare Terraform Provider version 4 to v5?
No. You’ll always have to follow the upgrade guide. If you don’t use modules, Cloudflare provides an automated update path. If you do, you can find hints in this article.
Why did Cloudflare rewrite the Terraform provider in version 5?
As stated in their guide, Cloudflare switched to a method where the provider is auto-generated from OpenAPI specifications, resulting in a different naming and attribute scheme.
Is there an automated way to migrate cloudflare_record to cloudflare_dns_record?
You can use the script provided in this article for an semi-automated approach to upgrade cloudflare_record resources to cloudflare_dns_record.
Other english articles
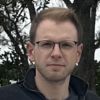
M.Sc. in Business Informatics and IT Consultant
I am Pascal, IT developer and consultant from Cologne, specialized in cloud infrastructure and DevOps. In my free time I like to travel, do sports or deal with technology, SmartHome or other nerd topics 🙂